What is the difference between Direct3D 11 and prior graphics APIs like Direct3D 9 or 10? What are the improvements Direct3D 11 has over previous versions?
DirectX 11 is a set of multimedia APIs by Microsoft, primarily focused on 3D graphics with Direct3D 11, audio, input, and display management. It introduces features like tessellation, multi-threading, and compute shaders, optimizing performance for modern GPUs on Windows.
In this blog, I have provided comparison between Direct3D 11 and prior graphics APIs.
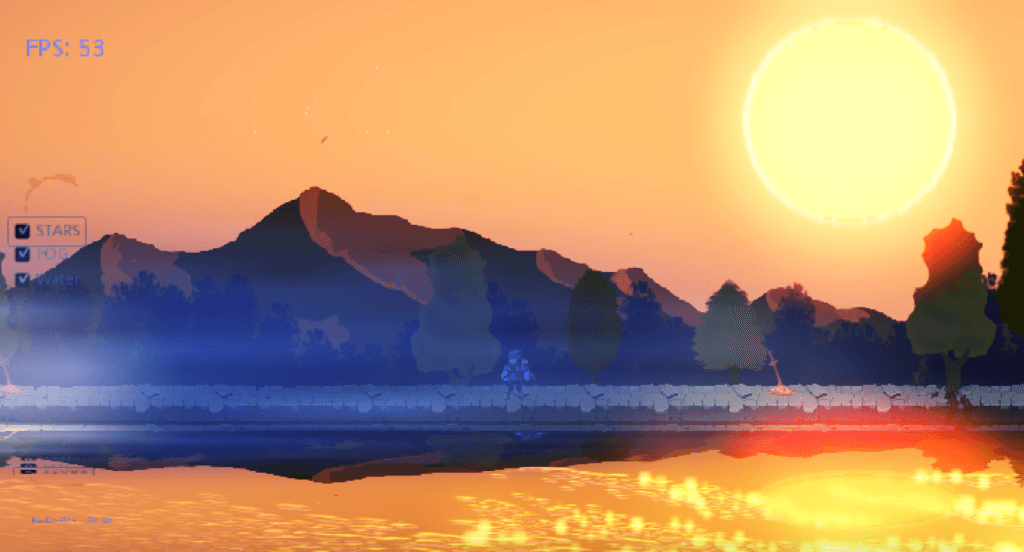
What are the key differences between Direct3D 9 and Direct3D 11?
Feature | Direct3D 9 | Direct3D 11 |
---|---|---|
Shader Model | Shader Model 2.0 / 3.0 | Shader Model 5.0 (supports Compute & Tessellation Shaders) |
Pipeline Type | Fixed-function pipeline with programmable shaders | Fully programmable pipeline with no fixed-function transformations |
Multi-threading | No built-in multi-threading support | Introduces deferred contexts for multi-threading efficiency |
Tessellation | Not supported | Supports Hull, Domain, and Tessellation Shaders |
Resource Management | Explicit memory management | More advanced resource management with views (SRV, UAV, RTV) |
Compute Shaders | Not available | Supports Compute Shaders for general-purpose GPU computing (GPGPU) |
Instancing | Limited support | Improved instancing support for rendering multiple objects efficiently |
DXGI (Swap Chain & Presentation) | Handled within Direct3D | Uses DXGI for display management, making Direct3D more modular |
Driver Model | Windows XP Driver Model (XDDM) | Windows Display Driver Model (WDDM), which improves efficiency and stability |
How does Direct3D 11 improve multi-threading support compared to Direct3D 10?
In Direct3D 10, all rendering commands had to be issued from a single thread, which could create CPU bottlenecks in multi-threaded applications. Direct3D 11 introduced several improvements to support multi-threading:
- Deferred Contexts
- Allows multiple threads to record command lists independently.
- These command lists can then be executed by the immediate context on the main thread.
- Helps in scaling CPU-side work across multiple cores.
- Command Lists
- Commands recorded in a deferred context can be stored as command lists.
- The main thread can execute these lists asynchronously, reducing CPU workload.
- Thread-Safe Resource Creation
- Allows resources (textures, buffers, shaders) to be created on multiple threads concurrently.
- Asynchronous Compute (Compute Shaders)
- Supports compute shaders that can run independently from rendering, maximizing GPU utilization.
These improvements allow applications to better utilize multi-core CPUs by distributing rendering work across multiple threads, leading to better performance and reduced CPU bottlenecks.
What are the main components of a Direct3D 11 rendering pipeline?
The Direct3D 11 rendering pipeline consists of several programmable and fixed-function stages:
- Input Assembler (IA) Stage
- Reads vertex data from buffers.
- Assembles primitives (triangles, lines, points).
- Vertex Shader (VS)
- Processes each vertex individually.
- Transforms vertices from object space to clip space.
- Outputs vertex position and attributes for the next stage.
- Hull Shader (HS) (Optional, for tessellation)
- Processes patches of control points.
- Outputs tessellation factors for the next stage.
- Tessellator (TESS) (Optional)
- Subdivides geometry based on tessellation factors.
- Generates additional vertices dynamically.
- Domain Shader (DS) (Optional, for tessellation)
- Processes newly generated vertices from the tessellator.
- Computes final vertex positions.
- Geometry Shader (GS) (Optional)
- Operates on entire primitives (e.g., triangles, lines).
- Can generate additional geometry dynamically.
- Stream Output (SO) (Optional)
- Allows geometry to be written to buffers for later use (e.g., particle systems).
- Rasterizer (Fixed-Function)
- Converts geometry into pixels.
- Performs clipping and viewport transformation.
- Pixel Shader (PS)
- Executes once per pixel.
- Determines the final color of each pixel.
- Output Merger (OM) (Fixed-Function)
- Blends pixel shader outputs with existing framebuffer contents.
- Applies depth/stencil testing and alpha blending.
- Compute Shader (CS) (Optional, GPGPU Compute)
- A separate parallel execution pipeline for general-purpose GPU computations.
Explain the role of ID3D11Device and ID3D11DeviceContext. How are they different?
ID3D11Device
- Represents the Direct3D 11 device (hardware abstraction).
- Responsible for creating GPU-related resources (textures, buffers, shaders).
- Typically a long-lived object (created once and reused).
ID3D11DeviceContext
- Represents the rendering context (command submission).
- Handles issuing draw calls, binding resources, and executing commands.
- Can be of two types:
- Immediate Context: Executes rendering commands in real-time.
- Deferred Context: Records commands for later execution (multi-threading support).
Key Differences
Feature | ID3D11Device | ID3D11DeviceContext |
---|---|---|
Purpose | Creates and manages GPU resources | Issues rendering commands |
Thread Safety | Thread-safe (can be used across threads) | Only the immediate context is thread-safe |
Lifetime | Typically created once per application | Can be created per-thread for multi-threading |
Type of Work | Resource creation, capability queries | Drawing, state management, compute dispatch |
Example creation of Direct3D 11 device and device context
// Create the Direct3D 11 device and device context
ID3D11Device* device = nullptr;
ID3D11DeviceContext* context = nullptr;
D3D11CreateDevice(
nullptr, // Default adapter
D3D_DRIVER_TYPE_HARDWARE, // Use GPU hardware acceleration
nullptr, // No software rasterizer
0, // No debug flags
nullptr, 0, // Default feature levels
D3D11_SDK_VERSION,
&device, // Output device
nullptr, // Feature level (optional)
&context // Output immediate context
);
// Use device to create a buffer
ID3D11Buffer* vertexBuffer = nullptr;
D3D11_BUFFER_DESC bufferDesc = {};
bufferDesc.Usage = D3D11_USAGE_DEFAULT;
bufferDesc.ByteWidth = sizeof(float) * 9; // Example size
bufferDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
device->CreateBuffer(&bufferDesc, nullptr, &vertexBuffer);
// Use context to bind and draw
UINT stride = sizeof(float) * 3;
UINT offset = 0;
context->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
context->Draw(3, 0); // Draw a triangle