In this blog, we will learn how to start and stop an AWS EC2 instance using Python boto3 scripts. This can be useful if you have an application that needs to start and stop an instance on a regular basis or if you want to automate the process of starting and stopping instances to save on costs.
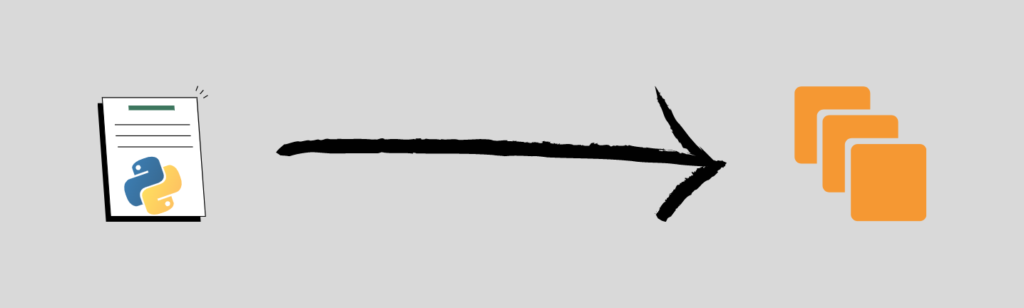
Introduction:
AWS, or Amazon Web Services is a cloud based computing platform that offers a range services including storage, computing, and networking. Among these services is Elastic Compute Cloud (EC2) which enables users to rent virtual machines for running their web applications, known as instances.
Prerequisites:
- Python 3
- AWS account
- AWS CLI (Command Line Interface) installed and configured
Step 1: Import the necessary libraries
To start an AWS instance using a Python script, we will need to import the boto3
library. Boto3 is a Python library that allows you to communicate with AWS services, including EC2.
import boto3
Step 2: Connect to AWS EC2
Next, we need to create a connection to the EC2 service. To do this, we will use the boto3
client for EC2.
ec2 = boto3.client('ec2', aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_ACCESS_KEY')
You will get the ACCESS_KEY and SECRET_ACCESS_KEY from AWS dashboard. You can follow these steps mentioned on AWS website.
Step 3: Start an instance
To start an instance, we will use the start_instances
method of the EC2 client. This method takes a list of instance IDs as an argument and returns a list of InstanceId
objects.
response = ec2.start_instances(InstanceIds=['INSTANCE_ID'])
Replace INSTANCE_ID
with the ID of the instance that you want to start.
Step 4: Stop an instance
To stop an instance, we will use the stop_instances
method of the EC2 client. This method also takes a list of instance IDs as an argument and returns a list of InstanceId
objects.
response = ec2.stop_instances(InstanceIds=['INSTANCE_ID'])
Again, replace INSTANCE_ID
with the ID of the instance that you want to stop.
Sample script
Here are sample scripts:
import boto3
# Replace YOUR_ACCESS_KEY and YOUR_SECRET_ACCESS_KEY with your actual
# access key and secret access key
client = boto3.client('ec2', aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_ACCESS_KEY')
# Replace INSTANCE_ID with the actual ID of the instance you want to start
response = client.start_instances(InstanceIds=['INSTANCE_ID'])
print(response)
import boto3
# Replace YOUR_ACCESS_KEY and YOUR_SECRET_ACCESS_KEY with your actual
# access key and secret access key
client = boto3.client('ec2', aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_ACCESS_KEY')
# Replace INSTANCE_ID with the actual ID of the instance you want to stop
response = client.stop_instances(InstanceIds=['INSTANCE_ID'])
print(response)
Conclusion:
In this blog, I have shown how to start and stop an AWS instance using Python scripts. We used the boto3
library and the EC2 client to connect to the EC2 service and start or stop an instance using the start_instances
and stop_instances
methods. Using this script, you can automate the process of starting and stopping instances to save on costs or integrate it into your application.